Alright, buckle up, cause I’m about to walk you through my little coding adventure: turning “100” into “91”. Sounds simple, right? Well, let me tell you, it was a bit of a journey.
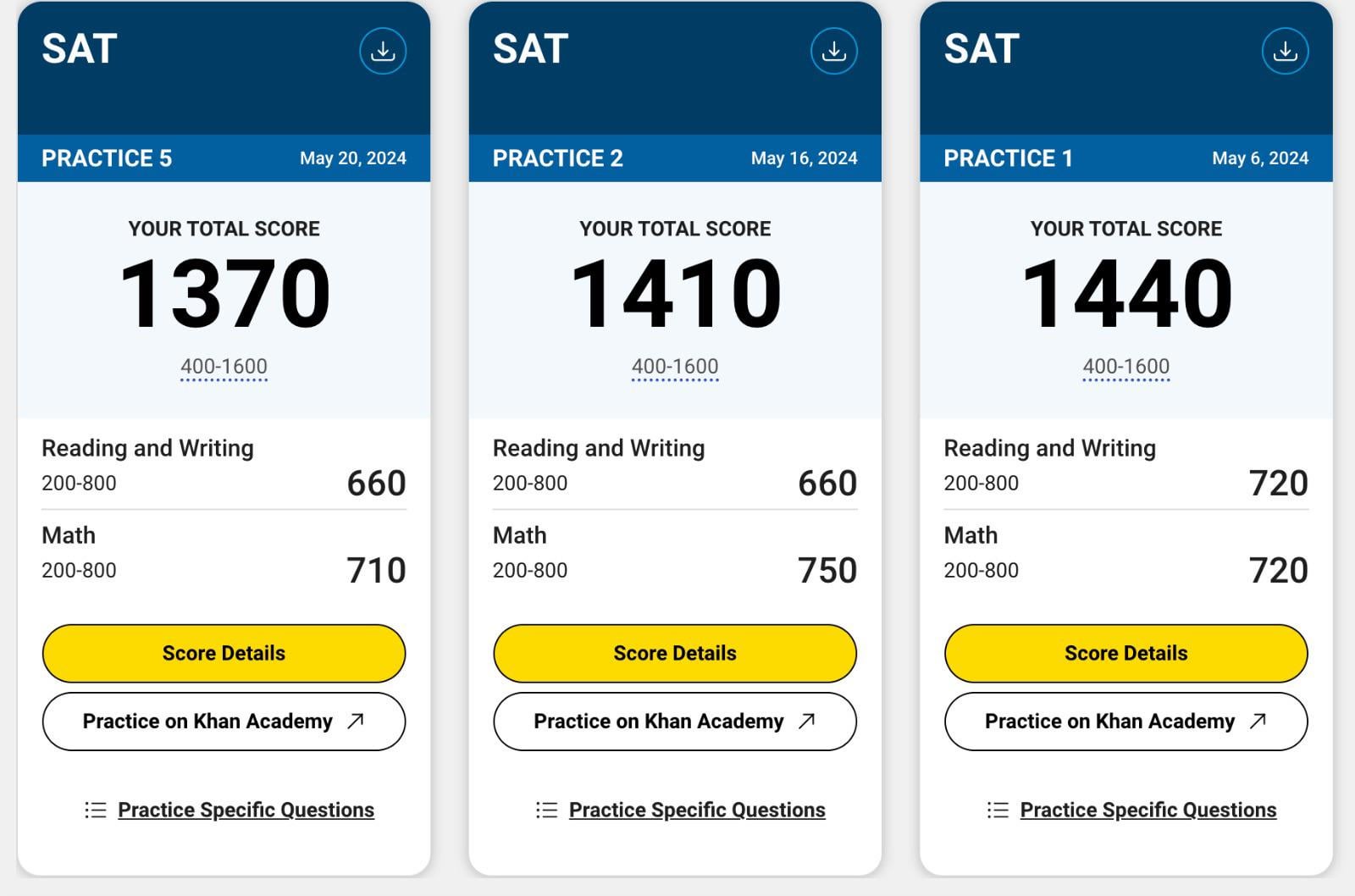
So, it all started when I needed to figure out a way to, you know, subtract 9 from 100, but in a roundabout way. I couldn’t just do 100 – 9. That’s too easy. I wanted to make things interesting.
First off, I grabbed my trusty IDE and started a new project. Nothing fancy, just a basic setup to get my hands dirty. The goal was clear: take the number 100 and, through a series of steps, end up with 91.
- I initially thought about using loops. Maybe I could start with 100 and decrement it one by one, nine times. Seemed straightforward, but kinda boring.
Then, I had a brighter idea. What if I used some multiplication and division to make the process more convoluted? Sounds fun, right?
I declared a variable named ‘number’ and initialized it to 100.
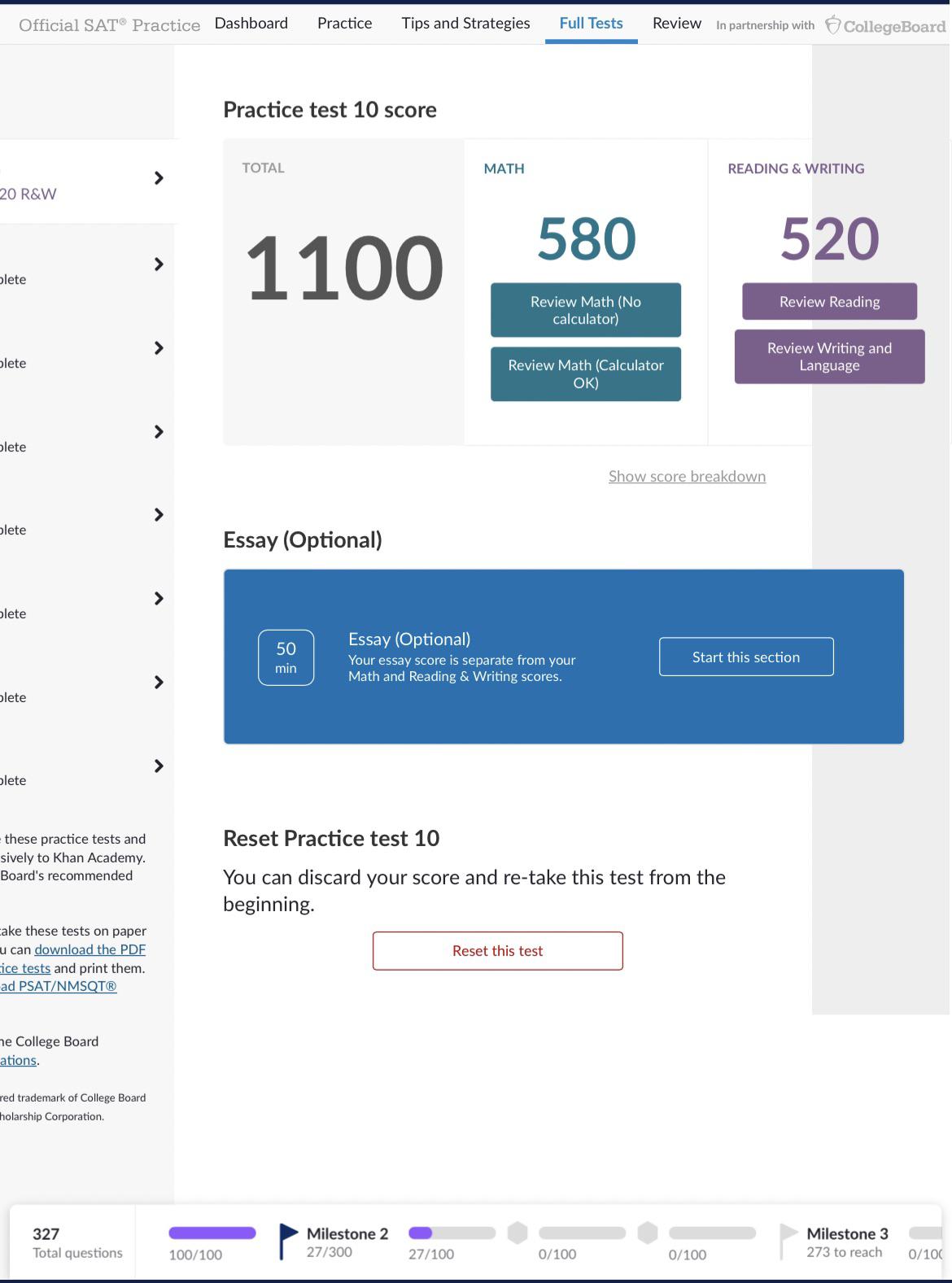
Here’s what I did:
- I figured, let’s multiply 100 by 2. Bam! `number = number 2;` Now we’re at 200.
- Next, I divided that by 2. `number = number / 2;` Back to 100. I know, I know, it seems pointless, but trust me, it was part of the plan.
- Now, let’s get serious. I decided to subtract a magic number and added another number to it. `number = number – 5;`
- Then to get close to 91 I subtracted another number. `number = number – 4;`
Okay, so at this point, the number was 91. Mission accomplished! But where’s the fun in stopping there? I felt like adding a bit of extra flair.
I decided to throw in a conditional statement just for kicks.
pseudocode
if (number == 91) {
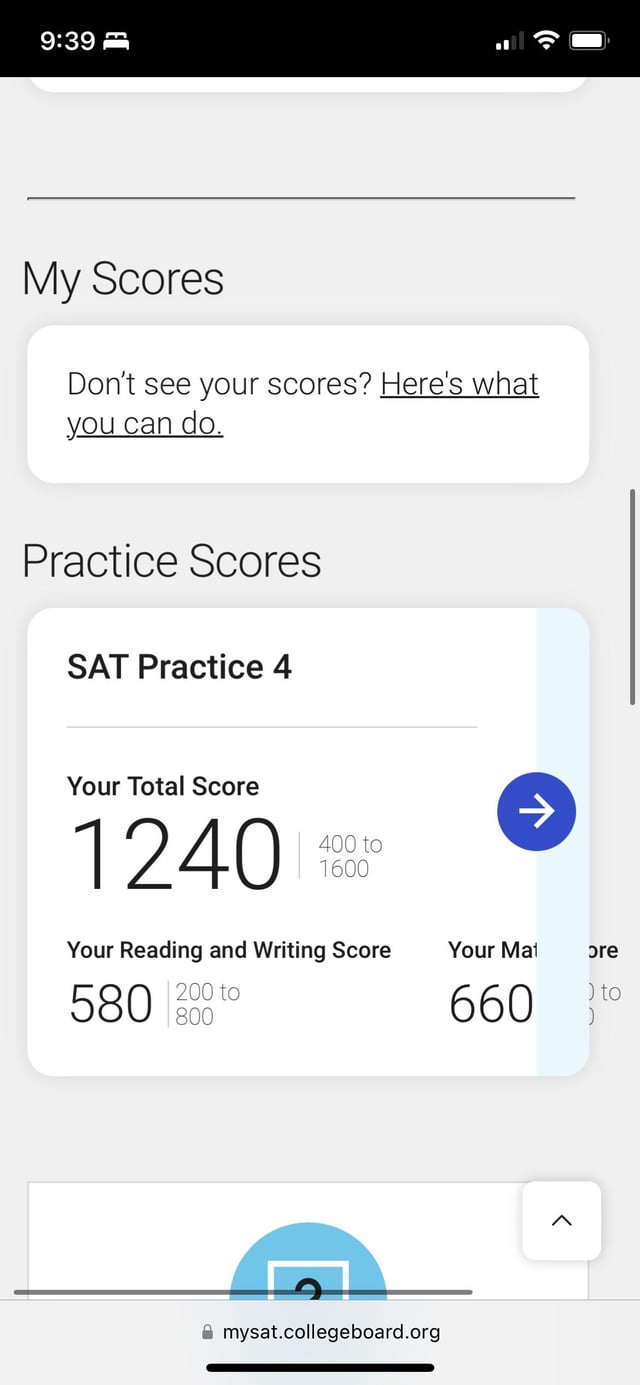
print(“We did it! Number is 91.”);
} else {
print(“Something went wrong.”);
And guess what? It printed “We did it! Number is 91.” Victory!
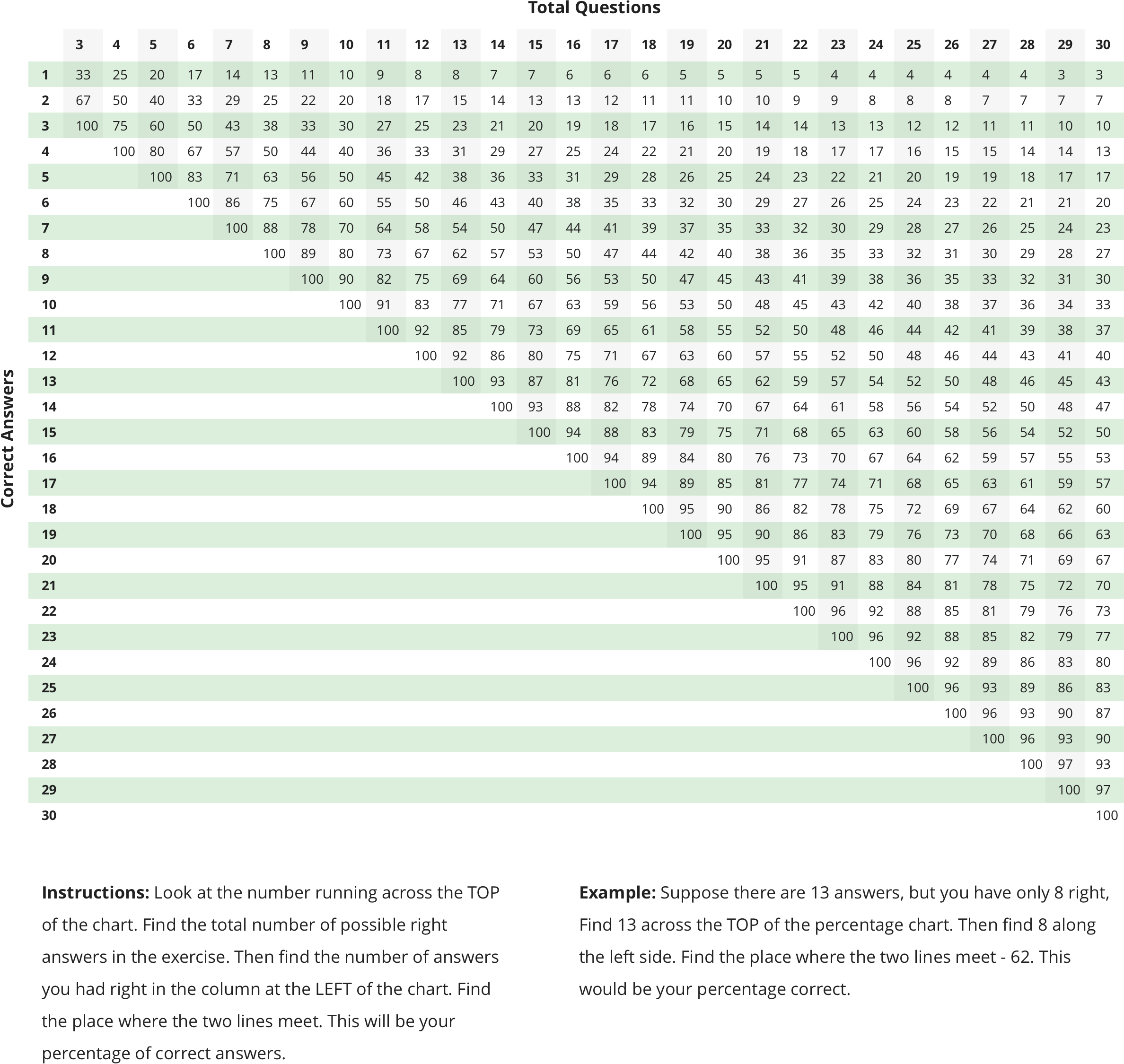
Looking back, it was a silly little exercise, but it was a good reminder of how many different ways there are to solve a problem. And sometimes, the most complicated solution is the most fun to implement.
It’s a bit like using a Rube Goldberg machine to turn on a light switch. Totally unnecessary, but oh-so-satisfying when it finally works.