Okay, so today I’m gonna talk about this thing called “tf split”. I was working on a project, and I needed to chop up some data, right? So I was like, “How do I do this?” And then I stumbled upon this “tf split” thing in TensorFlow. It’s like a function, or whatever, that lets you split up a tensor into smaller chunks.
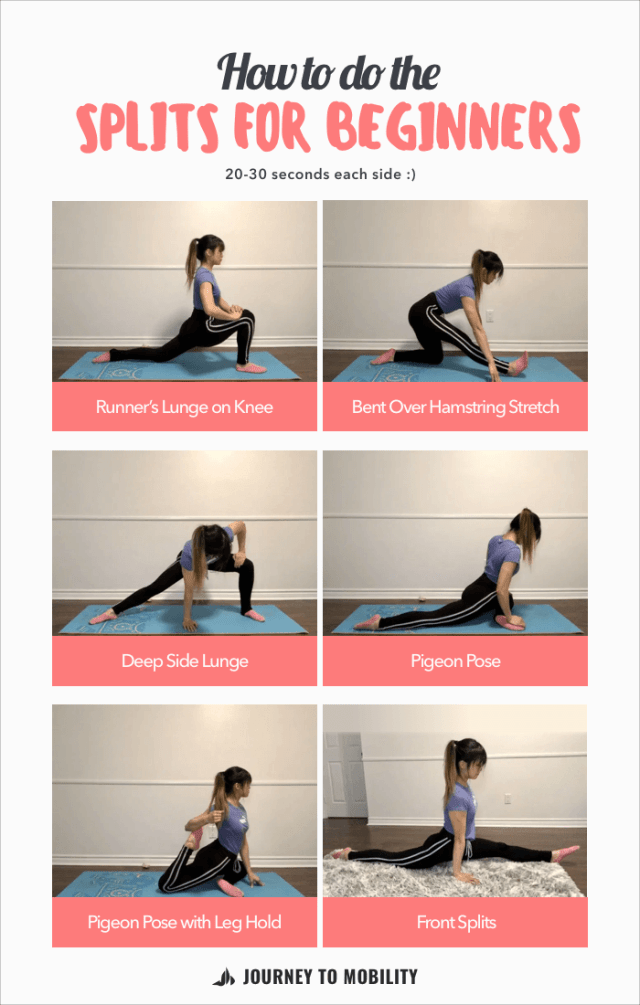
First, I had to install TensorFlow. I went to the command prompt, and I just typed pip install tensorflow. Easy as pie. Then, I fired up my Python editor, and I started to code, first I had to import TensorFlow, and I don’t know why we always name it ‘tf’. Anyway, I typed import tensorflow as tf. There you go.
Next, I had to create a tensor. Now, this is just a fancy word for an array, or a matrix, you know? So I made a simple one, I typed something like my_tensor = *([[1, 2, 3], [4, 5, 6], [7, 8, 9]]). That’s a 3×3 matrix, nothing special.
Then came the fun part, the splitting. I used the function. You gotta tell it what tensor you wanna split, how many pieces you want, and along which axis. So, I wanted to split my tensor into three parts along the first axis (the rows). I typed split_tensors = *(my_tensor, num_or_size_splits=3, axis=0). Boom! Done.
After that, I wanted to see what I got, so I used print() function for each of them. like:
- print(split_tensors[0])
- print(split_tensors[1])
- print(split_tensors[2])
Check the result
And there you have it! I got three separate tensors, each with one row from the original tensor. It’s like magic! I mean, it’s not really magic, it’s just code, but it feels like it, you know?
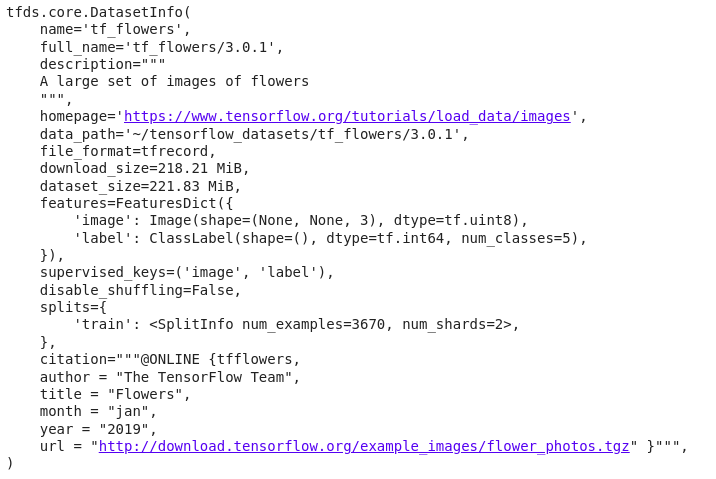
So yeah, that’s how I used “tf split”. It’s pretty handy when you’re dealing with large datasets and you need to break them down into smaller, more manageable parts. I’m not a TensorFlow expert or anything, but I managed to figure it out, so you can too! Just remember to install TensorFlow first. It was a bit of a hassle, but I did it in the end.
It’s not rocket science, just a bit of trial and error. I messed up a few times, got some error messages, but I kept at it. You know how it is. Anyway, it’s a neat little tool to have in your arsenal, so give it a try if you ever need to split some tensors. It makes things a whole lot easier.
Hope this helps someone out there!