Okay, so today I’m gonna walk you through how I put together a 2010 World Cup table. It’s not super complicated, but I figured someone might find it useful, or at least mildly entertaining.
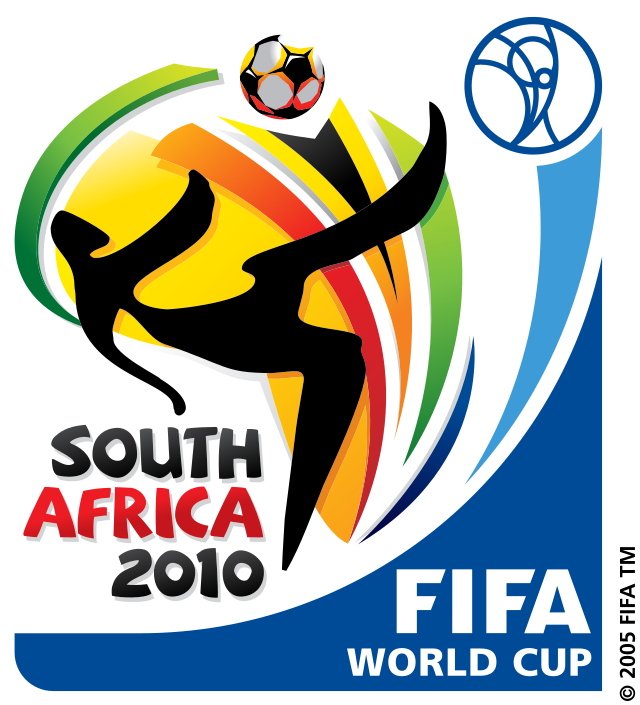
First off, I started by gathering the data. I just grabbed it from a sports website, copy-pasted all the match results into a text file. It was messy, like REALLY messy. Think random spaces, weird characters, the whole nine yards.
Then came the data cleaning. Ugh. I fired up my text editor and started going through it line by line. Replaced tabs with commas, got rid of extra spaces, made sure the scores were in the right format (like, team A goals – team B goals). This took way longer than I thought it would.
Next up: deciding on a data structure. I thought about using a dictionary, but a list of lists seemed simpler for what I wanted to do. Each inner list would represent a team, and would have their name, games played, wins, losses, draws, goals for, goals against, and points. Pretty standard stuff.
Now for the fun part (sort of): writing the code. I started by creating an empty list called “table”. Then I looped through the cleaned-up match results. For each match, I split the string into team names and scores. I had to be careful here to handle cases where the team names had spaces in them.
Inside the loop, I had to update the team stats. First, I checked if each team was already in the “table” list. If not, I added them with all the initial values set to zero (except the team name, of course). Then, I incremented the “games played” count for both teams. Based on the score, I incremented the “wins”, “losses”, or “draws”, and updated the “goals for” and “goals against”.
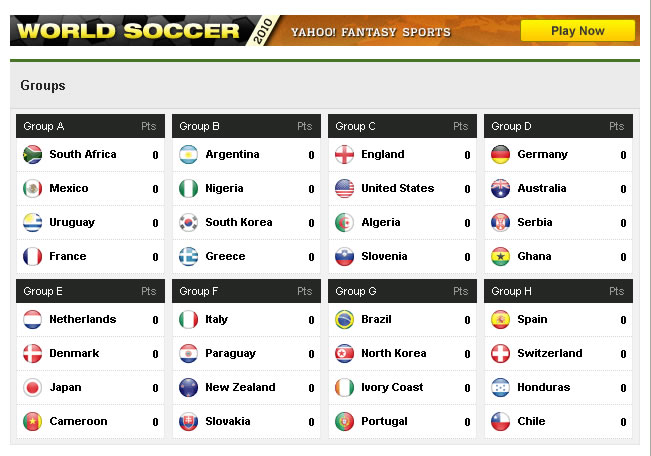
Calculating points was easy: 3 points for a win, 1 for a draw, 0 for a loss. I just added that to the team’s “points” value.
After processing all the matches, I had a list of lists with all the team stats. But it was in a random order. Time to sort the table. I used the `sort()` method with a custom key function. I sorted first by points (descending), then by goal difference (goals for minus goals against, also descending), and finally by goals for (descending again). This ensured that the teams were ranked according to the official FIFA rules.
Finally, I printed the table. I used a `for` loop to iterate through the sorted list, and formatted the output nicely. I made sure the columns were aligned, and added a header row with the column names.
The trickiest part? Dealing with the messy data and getting the sorting right. I spent a good chunk of time debugging those two things. I also had a brain fart moment where I forgot to initialize the team stats to zero, which led to some very weird results.
What I learned? Data cleaning is a pain, but it’s essential. Also, thinking carefully about the data structure and the sorting logic can save a lot of headaches later on. And always, ALWAYS double-check your initialization!
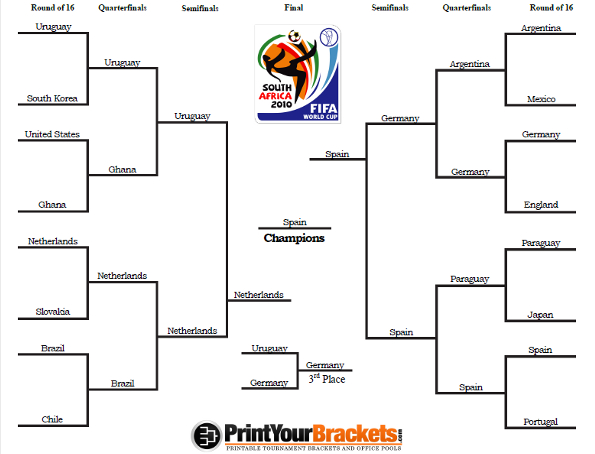
Here’s a simplified overview of the steps:
- Get the data
- Clean the data
- Choose a data structure
- Write code to process matches and update team stats
- Sort the table
- Print the table
That’s pretty much it. Nothing too fancy, but it got the job done. Now I have a nice, clean 2010 World Cup table that I can use for whatever reason I might need it for.