Alright, let’s talk about that “calendar 2008” thing I messed around with. It was a while back, but the memories are still kinda fresh, you know?
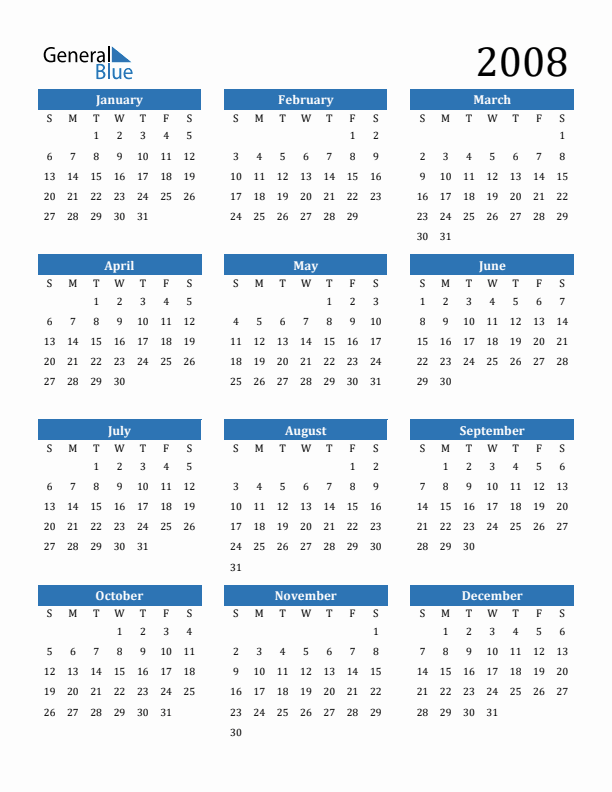
The Idea Sparked: So, I was fiddling with some Python stuff, right? Needed a quick way to display a calendar for a specific year. Looked around, didn’t find anything exactly what I wanted, so I figured, “Why not build my own?” Classic developer move, I guess.
Getting Started: First things first, I fired up my trusty text editor (probably Sublime Text back then, maybe even Notepad++, who knows?). I started a new Python file, something like calendar_*. The initial plan was super simple:
- Figure out how to get the day of the week for January 1st, 2008.
- Print out the days of the week in a nice header: Sun Mon Tue Wed Thu Fri Sat
- Loop through the days, printing the numbers, keeping track of when to start a new line for each week.
- Handle different month lengths (28, 29, 30, 31 days).
Diving into the Code: I remember wrestling with the datetime
module in Python. It’s powerful, but sometimes feels like you need a PhD to use it properly. I used it to grab the weekday of January 1st. Something like:
import datetime
first_day = *(2008, 1, 1).weekday()
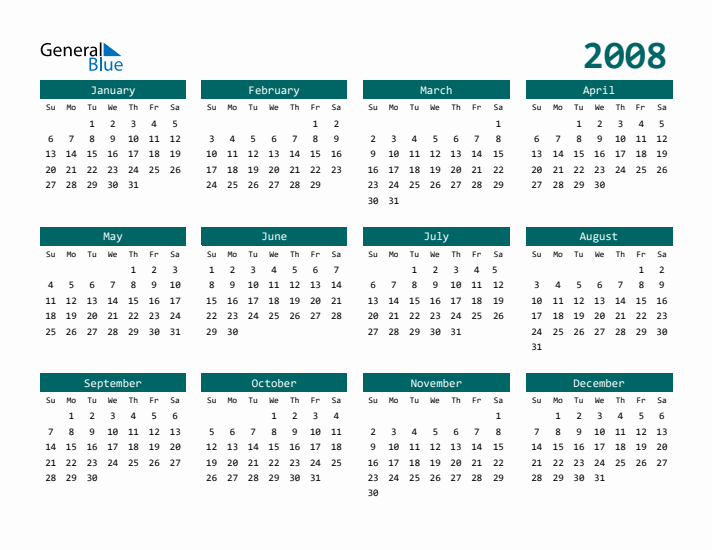
That .weekday()
thing returns a number (0 for Monday, 6 for Sunday). So, I had to figure out how many spaces to print before the “1” on the calendar.
The Printing Logic: This was the tricky part. I needed to keep track of the current day of the week and the current day of the month. Lots of if
statements, I tell ya. Something along these lines:
print("Sun Mon Tue Wed Thu Fri Sat")
for i in range(first_day):
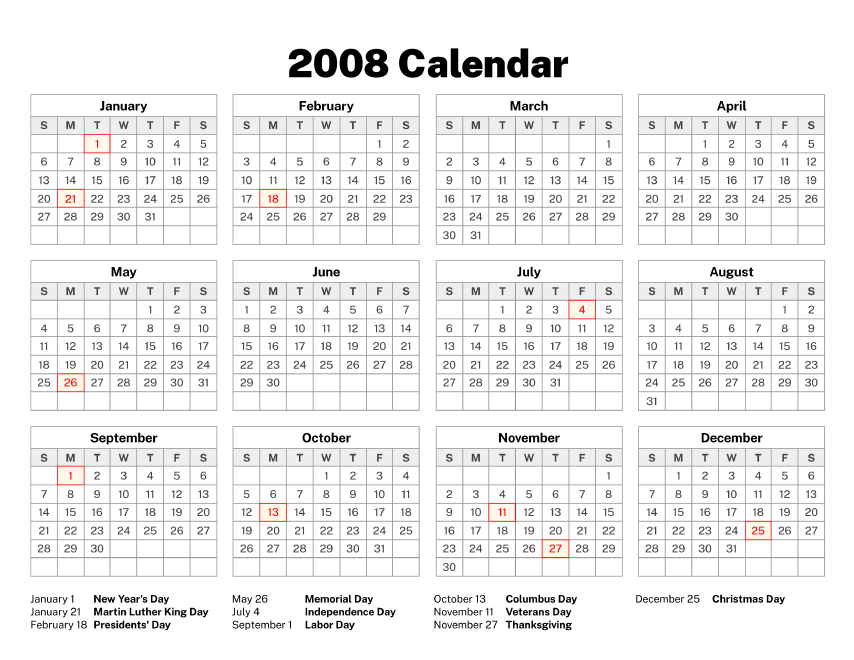
print(" ", end="") # Four spaces for alignment
day_of_month = 1
day_of_week = first_day
while day_of_month <= days_in_month:
print(f"{day_of_month:3} ", end="") # Format for alignment
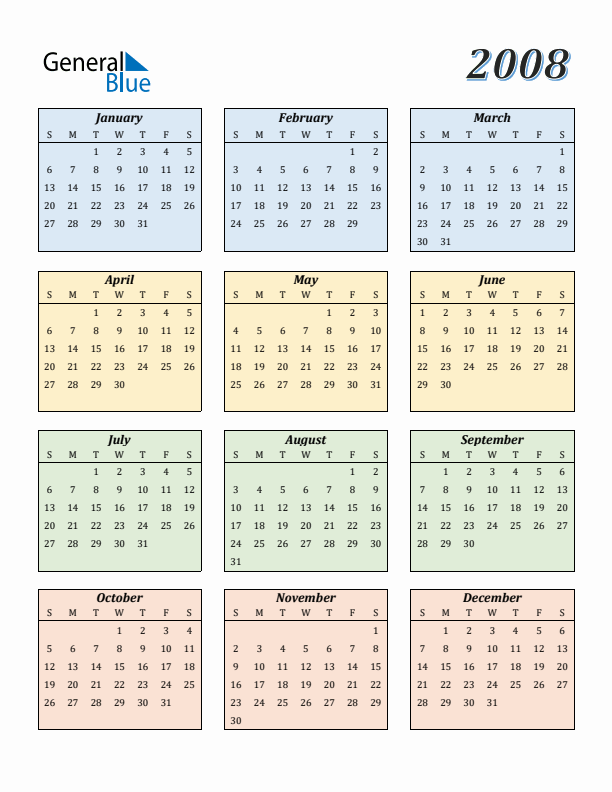
day_of_month += 1
day_of_week = (day_of_week + 1) % 7
if day_of_week == 0:
print() # New line for Sunday
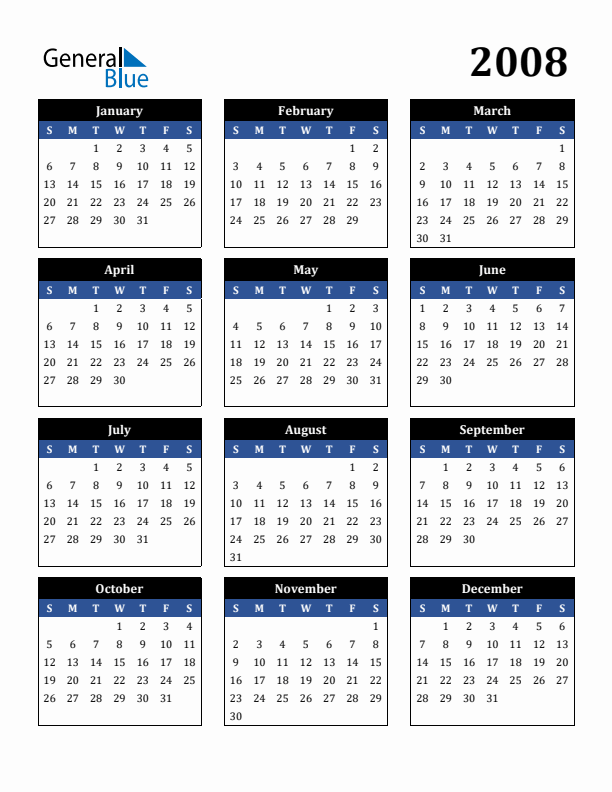
Handling Months: Getting the right number of days for each month was another fun challenge. I didn’t want to hardcode it, so I used a list or dictionary (can’t remember exactly) to store the number of days in each month. Plus, gotta remember February and those pesky leap years!
Leap Year Shenanigans: 2008 was a leap year, so February had 29 days. Had to add a check for that. Something like:
def is_leap_year(year):
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
return True
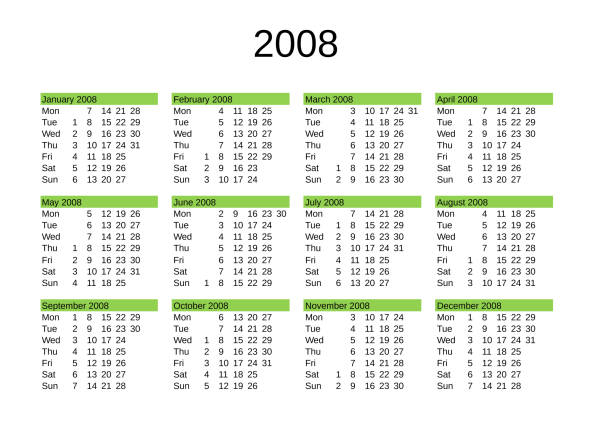
else:
return False
Putting it All Together: I wrapped all that month logic into a loop that iterated through each month of the year. Called my printing function for each month, passing in the month number and the number of days in that month.
The Output: After a bunch of debugging (and probably some frustrated yelling at the screen), I finally got it to print out a decent-looking calendar for 2008. It wasn’t fancy, just plain text in the terminal, but it worked!
What I Learned: It was a good exercise in using the datetime
module and just basic programming logic. Learned a bit more about how calendars actually work and how to manipulate dates in Python. Plus, it was kinda satisfying to build something from scratch, even if it was something relatively simple.
Would I Do It Again? Probably not. There are plenty of calendar libraries out there that do a much better job. But hey, it was a fun little project at the time!